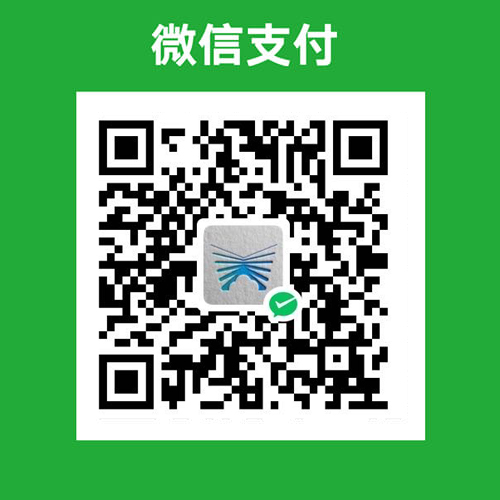
第6课 选择结构-if-else if
发布时间:
一、if-else if
语法格式:
if (condition1) {
// if语句执行的代码块1
} else if (condition2) {
// else if语句执行的代码块2
} else if (condition3) {
// else if语句执行的代码块3
} else {
// if语句执行的代码块4
}
其中,condition1、condition2、condition3等是一系列条件表达式,它们的值都是布尔型(true或false)。如果condition1的值为true,那么if语句执行代码块1中的语句;如果condition1的值为false,那么程序会执行第一个else if语句,并检查condition2的值。如果condition2的值为true,则程序执行代码块2中的语句;否则程序继续检查下一个else if语句。如果所有的else if语句都不满足条件,那么程序会执行最后一个else语句中的代码块4。
下面是一个使用if-else if语句实现的简单程序,该程序根据用户输入的分数输出相应的等级:
int main() {
int score = 0;
cout << "请输入考试分数:” << endl;
cin >> score;
if (score > 600){
cout<< "我考上了一本大学”<<endl;
}else if (score > 500) {
cout<< “我考上了二本大学” <<endl;
}else if (score > 400) {
cout <<量我考上了三本大学”<<endl;
}else {
cout < <“我未考上本科”<< endl;
}
return 0;
}
例1:
假设某快递公司的计费标准如下:
● 不超过2kg,每kg 10元;
● 超过2kg但不超过5kg,2kg内每kg 10元,超过2kg部分每kg 8元;
● 超过5kg,2kg内每kg 10元,3kg~5kg内每kg 8元,超过5kg部分每kg 5元。
#include <iostream>
using namespace std;
int main() {
double weight, price;
cout << "Please input the weight of the package: ";
cin >> weight;
if (weight <= 2) {
price = weight * 10;
} else if (weight <= 5) {
price = 2 * 10 + (weight - 2) * 8;
} else {
price = 2 * 10 + 3 * 8 + (weight - 5) * 5;
}
cout << "The price of the package is " << price << " yuan." << endl;
return 0;
}
例2 输出绝对值
例3 判断数正负
二、if嵌套
C++中的if嵌套是指在一个if语句的分支语句中,再嵌套一个if语句。其主要作用是根据多个条件的组合来进行复杂的判断。 if嵌套的语法格式如下:
if (条件1) {
// 条件1成立时执行的语句
if (条件2) {
// 条件1和条件2都成立时执行的语句
}
else {
// 条件1成立但条件2不成立时执行的语句
}
}
else {
// 条件1不成立时执行的语句
}
可以看出,if嵌套语句中的每一个if语句都可以带有自己的else分支。在实际应用中,if嵌套可以进行多层嵌套,根据实际情况进行组合。
例3: 以下是一个使用if嵌套实现的例题,题目为:输入一个年份和月份,输出该月份的天数。
#include <iostream>
using namespace std;
int main() {
int year, month;
cout << "请输入年份和月份:" << endl;
cin >> year >> month;
if (month == 2) {
if ((year % 4 == 0 && year % 100 != 0) || year % 400 == 0) {
cout << "本月有29天" << endl;
} else {
cout << "本月有28天" << endl;
}
}else if (month == 4 || month == 6 || month == 9 || month == 11) {
cout << "本月有30天" << endl;
}else {
cout << "本月有31天" << endl;
}
return 0;
}
例4: 简单计算器
#include<iostream>
using namespace std;
int main(){
int a,b;
char c;
cin>>a>>b>>c;
if(c=='+'){
cout<<a+b;
}else if(c=='-'){
cout<<a-b;
}else if(c=='*'){
cout<<a*b;
}else if(c=='/'){
if(b==0){
cout<<"Divided by zero!";
}else{
cout<<(double)a/b;
}
}else{
cout<<"Invalid operator";
}
return 0;
}
三、综合理解
**例5:**使用if嵌套的格式,完成三个数从小到大排序。
有三只小猪ABC,请分别输入三只小猪的体重,并旦判断哪只小猪最重
#include <iostream>
using namespace std;
int main() {
int a, b, c, temp;
cout << "请输入三个整数:" << endl;
cin >> a >> b >> c;
if (a <= b) {
if (a <= c) {
if (b <= c) {
// a <= b <= c
cout << "从小到大排序后的结果为:" << a << " " << b << " " << c << endl;
} else {
// a <= c < b
cout << "从小到大排序后的结果为:" << a << " " << c << " " << b << endl;
}
} else {
// c < a <= b
cout << "从小到大排序后的结果为:" << c << " " << a << " " << b << endl;
}
} else {
if (b <= c) {
if (a <= c) {
// b <= a <= c
cout << "从小到大排序后的结果为:" << b << " " << a << " " << c << endl;
} else {
// b <= c < a
cout << "从小到大排序后的结果为:" << b << " " << c << " " << a << endl;
}
} else {
// c < b < a
cout << "从小到大排序后的结果为:" << c << " " << b << " " << a << endl;
}
}
return 0;
}
**例6:**使用if-else if的形式,完成三个数从小到大排序。
#include <iostream>
using namespace std;
int main() {
int a, b, c;
cout << "请输入三个数:";
cin >> a >> b >> c;
if (a <= b && b <= c) {
cout << a << " " << b << " " << c << endl;
} else if (a <= c && c <= b) {
cout << a << " " << c << " " << b << endl;
} else if (b <= a && a <= c) {
cout << b << " " << a << " " << c << endl;
} else if (b <= c && c <= a) {
cout << b << " " << c << " " << a << endl;
} else if (c <= a && a <= b) {
cout << c << " " << a << " " << b << endl;
} else if (c <= b && b <= a) {
cout << c << " " << b << " " << a << endl;
}
return 0;
}
**例7:**使用if的形式,完成三个数从小到大排序,也是最简单的。
#include <iostream>
using namespace std;
int main() {
int a, b, c, temp;
cout << "请输入三个整数:" << endl;
cin >> a >> b >> c;
if (a > b) {
temp = a;
a = b;
b = temp;
}
if (a > c) {
temp = a;
a = c;
c = temp;
}
if (b > c) {
temp = b;
b = c;
c = temp;
}
cout << "从小到大排序后的结果为:" << a << " " << b << " " << c << endl;
return 0;
}